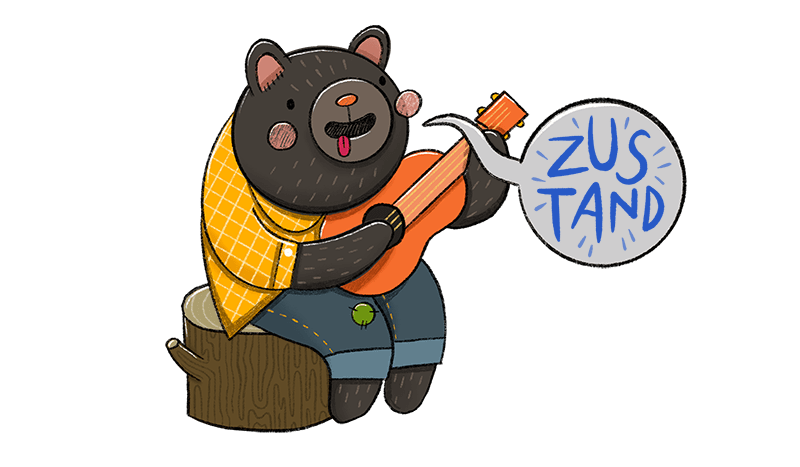
Zustand 🔥🔥🔥 Great!!!
With Zustand! It’s a lightweight state management library for React that is easy to use and quite performant. Here’s an overview:
What is Zustand?
Zustand is a small, fast, and scalable state management solution for React applications. It’s designed to be simple yet powerful enough to handle both small and large state needs. Zustand doesn’t rely on Context or reducers, making it more flexible and easier to use in many cases compared to other libraries like Redux.
Key Features of Zustand
- Minimal Boilerplate: Zustand provides a very simple API that requires little to no boilerplate.
- No Provider Component: Unlike Context API or Redux, Zustand doesn’t require wrapping your component tree in a provider component.
- Global and Local State Management: You can manage both global and local state with Zustand, giving you flexibility in how you structure your state management.
- Selectors and Performance: Zustand allows you to select specific slices of state, preventing unnecessary re-renders of components.
- Middleware Support: Zustand supports middleware like logging, persistence, and even async actions out of the box.
Example of Zustand Usage
-
Basic Store Setup:
import create from "zustand"; // Create a store const useStore = create((set) => ({ count: 0, increaseCount: () => set((state) => ({ count: state.count + 1 })), resetCount: () => set({ count: 0 }), })); // Usage in a component function Counter() { const { count, increaseCount, resetCount } = useStore(); return ( <div> <p>{count}</p> <button onClick={increaseCount}>Increase</button> <button onClick={resetCount}>Reset</button> </div> ); }
-
Selectors to Improve Performance: Zustand supports selecting specific parts of the state, reducing unnecessary re-renders when only certain state changes.
const count = useStore((state) => state.count); const increaseCount = useStore((state) => state.increaseCount);
-
Persisting State: Zustand supports persistence middleware, so your state can be persisted in local storage:
import create from "zustand"; import { persist } from "zustand/middleware"; const useStore = create( persist( (set) => ({ count: 0, increaseCount: () => set((state) => ({ count: state.count + 1 })), }), { name: "counter-storage" } // key for localStorage ) );
-
Async Actions: Zustand doesn’t natively support async actions like Redux with
redux-thunk
, but you can handle them easily using regular JavaScript async/await:const useStore = create((set) => ({ count: 0, fetchData: async () => { const response = await fetch("https://api.example.com/data"); const data = await response.json(); set({ count: data.count }); }, }));
Why Use Zustand?
- Simplicity: Less boilerplate compared to other state management solutions like Redux.
- Performance: Built-in optimizations like selectors reduce unnecessary renders.
- Flexibility: It works well for both small applications and larger apps with more complex state requirements.
- Scalability: You can start small with Zustand and gradually scale without much friction.
If you want to dive deeper into how you can use Zustand for your project or optimize it further, let me know!