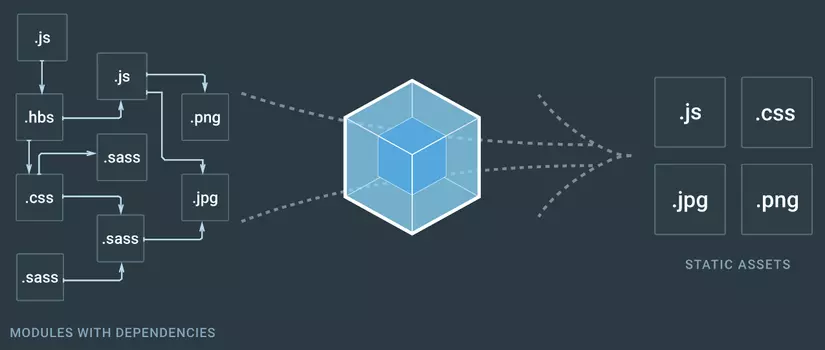
Webpack is a powerful and widely-used module bundler
Webpack is a powerful and widely-used module bundler for JavaScript applications. It takes all the modules (JavaScript files, styles, images, etc.) and bundles them into a single (or multiple) files that can be efficiently loaded by a browser. It is particularly useful for handling the complexity of large-scale JavaScript applications.
Key Features of Webpack:
Entry and Output:
-
Entry: The entry point is the starting point where Webpack begins building the dependency graph, typically your main JavaScript file.
-
Output: After bundling the assets, Webpack outputs the result to a file or folder, usually specified by the developer.
-
Example:
// webpack.config.js module.exports = { entry: './src/index.js', // The main entry file output: { filename: 'bundle.js', // The bundled file path: __dirname + '/dist', // Output directory } };
Loaders:
-
Webpack uses loaders to transform non-JavaScript files (like CSS, images, or TypeScript) into modules that can be bundled.
-
Loaders are configured in the Webpack configuration file and tell Webpack how to process files. Example of using a loader for CSS:
module.exports = { module: { rules: [ { test: /\.css$/, // Apply this rule to files ending in .css use: ['style-loader', 'css-loader'], // Use these loaders } ] } };
- css-loader interprets @import and url() like import/require() and resolves them.
- style-loader injects CSS into the DOM.
Plugins:
- Plugins extend Webpack’s functionality and handle tasks like optimizing the bundle, managing assets, and injecting environment variables.
- Some popular plugins include:
- HtmlWebpackPlugin: Automatically generates an HTML file that includes your bundle(s).
- TerserWebpackPlugin: Minifies JavaScript for production.
- MiniCssExtractPlugin: Extracts CSS into separate files.
- Example of using the HtmlWebpackPlugin:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html', // Use this HTML as a template
}),
],
};
Dev Server:
- Webpack offers a development server (webpack-dev-server) that automatically refreshes the browser when files change (hot-reloading).
- It provides a local server for testing during development.
- Example of using :
module.exports = {
devServer: {
contentBase: './dist', // Serve files from the dist directory
hot: true, // Enable hot module replacement (HMR)
},
};
Code Splitting:
- Code splitting is one of Webpack’s most powerful features. It allows you to split your code into various bundles, which can then be loaded on demand (lazy loading).
- This helps improve performance by loading only the necessary code when needed, rather than loading everything upfront.
Example of dynamic imports:
// Lazy load the `lodash` library
import(/* webpackChunkName: "lodash" */ 'lodash').then((_) => {
console.log(_.join(['Hello', 'Webpack'], ' '));
});
Tree Shaking:
- Webpack uses tree shaking to remove unused code from the final bundle. This is particularly useful when working with large libraries like Lodash or Moment.js.
- Tree shaking works best with ES modules (import/export syntax) as they allow for static analysis.
Asset Management:
- Webpack can handle static assets like images, fonts, and CSS.
- For example, using file-loader or url-loader, you can import images directly into your JavaScript files, and Webpack will bundle and optimize them.
Example:
module.exports = {
module: {
rules: [
{
test: /\.(png|jpg|gif)$/,
use: [
{
loader: 'file-loader',
options: {
name: '[name].[ext]', // Keep the original name and extension
outputPath: 'images/', // Output to the images folder
},
},
],
},
],
},
};
Example Webpack Configuration
Here’s a basic example of a webpack.config.js file:
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './src/index.js', // Entry point
output: {
filename: 'bundle.js', // Output bundle
path: path.resolve(__dirname, 'dist'), // Output directory
},
module: {
rules: [
{
test: /\.css$/, // Process CSS files
use: ['style-loader', 'css-loader'],
},
{
test: /\.(png|jpg|gif)$/, // Process images
use: ['file-loader'],
},
],
},
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html', // Generate HTML file
}),
],
devServer: {
contentBase: './dist', // Serve files from dist directory
hot: true, // Enable hot module replacement (HMR)
},
};
Webpack vs. Other Bundlers:
- Rollup: Rollup is typically used for bundling JavaScript libraries, focusing on smaller and more optimized bundles, while Webpack is more suited for large web applications with complex configurations.
- Parcel: Parcel offers a zero-config experience and is easier to set up for small projects, but Webpack is more flexible and feature-rich for larger and more complex setups.
Use Cases for Webpack:
- Single-Page Applications (SPAs): Webpack excels at bundling resources for SPAs. It efficiently bundles and optimizes assets, applies tree shaking, and supports code splitting for better performance.
- Managing Multiple Asset Types: Webpack is great when your project includes not only JavaScript but also CSS, images, and fonts. It can handle and optimize all these assets in one build pipeline.
- Development and Production Configurations: Webpack allows you to create separate configurations for development and production. You can include optimizations like minification and tree shaking in the production build while enabling features like hot-reloading in the development build.
Advantages of Webpack:
- Modularity: Handles all types of assets as modules (JavaScript, CSS, images, etc.).
- Extensibility: Plugins and loaders give Webpack extreme flexibility.
- Optimizations: Features like tree shaking, minification, and code splitting make it ideal for optimizing large applications.
- Hot Module Replacement (HMR): In development, Webpack can hot-reload modules without a full page refresh.
Disadvantages of Webpack:
- Complexity: Setting up Webpack can be daunting for new developers, especially for large projects with many moving parts.
- Configuration: While flexible, Webpack’s configuration can get verbose and complex compared to more minimal tools like Parcel.
Conclusion:
Webpack is a robust, feature-rich tool for bundling JavaScript applications. It provides flexibility and control over the build process, making it a go-to choice for large web applications, particularly SPAs. Its ecosystem of plugins and loaders allows developers to handle various types of assets, automate optimizations, and provide a smooth development experience.