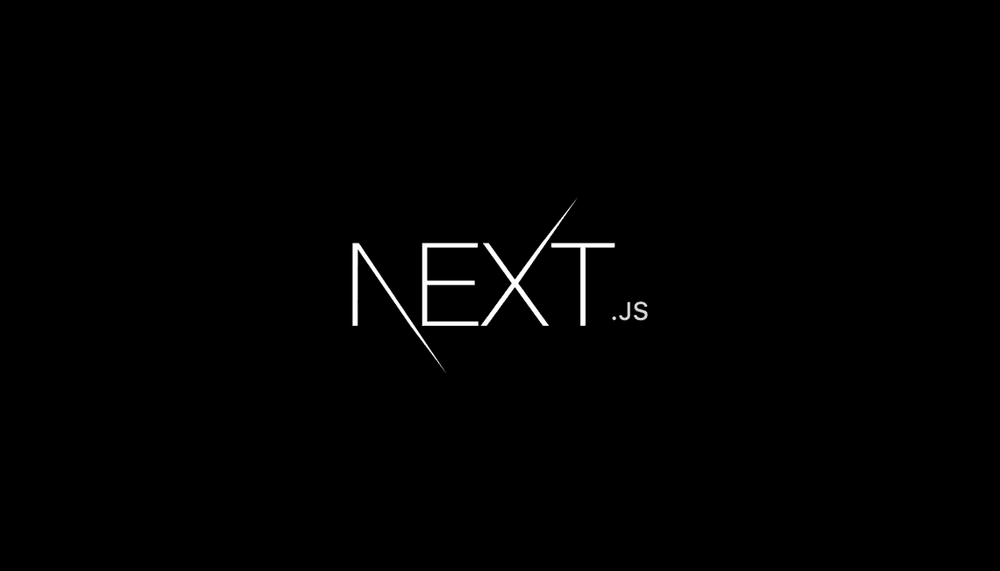
Is working on optimizing a React.js application using Next.JS
To optimize your React.js application using Next.js, here are some practical strategies you can implement:
1. Optimize Bundle Size
- Code Splitting: Automatically done by Next.js, but you can further enhance it by using dynamic imports (
next/dynamic
) for larger components or libraries that are not needed immediately. - Tree Shaking: Ensure only the code you use is included in the bundle by importing only specific functions or components from libraries.
- Analyze Bundle: Use the
next-bundle-analyzer
plugin to understand and minimize the size of your JavaScript bundles.
2. Optimize Images
- Use the
next/image
component for automatic image optimization with built-in lazy loading, responsive images, and image compression. - Implement a Content Delivery Network (CDN) to serve images faster.
3. Static Site Generation (SSG) & Incremental Static Regeneration (ISR)
- Use SSG to pre-render as many pages as possible at build time to speed up page loading.
- Utilize ISR to revalidate pages in the background and update them with new data without requiring a full rebuild.
4. Optimize API Calls
- Use SWR or React Query for client-side data fetching and caching to reduce redundant API calls and provide a smoother experience with data rehydration.
- Pre-fetch data at the server level using
getStaticProps
orgetServerSideProps
where needed.
5. Use Server-Side Rendering (SSR) Only When Necessary
- SSR can impact performance due to the additional server-side load. Use
getServerSideProps
sparingly and prefer SSG or client-side fetching whenever possible.
6. Prefetching Links and Resources
- Use
next/link
with theprefetch
option to pre-load routes for a smoother navigation experience.
7. Caching and Compression
- Utilize caching strategies with proper HTTP headers (e.g.,
Cache-Control
) to cache static assets, pages, and API responses. - Enable gzip or Brotli compression on the server to reduce the size of transferred files.
8. Optimize Fonts
- Use
next/font
for optimized Google Fonts loading with less layout shift and font preloading.
9. Improve Lighthouse Score
- Run a Lighthouse audit to analyze your app’s performance, accessibility, SEO, and best practices, then optimize based on its suggestions.
- Focus on reducing Largest Contentful Paint (LCP), First Input Delay (FID), and Cumulative Layout Shift (CLS) for a better Core Web Vitals score.
10. Lazy Load Components
- Use dynamic imports for components that are not immediately necessary, such as modal windows or carousels, to speed up the initial load time.
11. Optimize Third-Party Scripts
- Defer loading of third-party scripts like analytics, ads, or social media widgets. Only load them when necessary to prevent them from blocking the initial page rendering.
12. Consider Edge Functions
- Use Next.js Edge Functions to run server-side code closer to the user for faster page rendering and low-latency data fetching.
These approaches will help improve both performance and user experience for your Next.js application. If you have specific areas you’d like to optimize further, let me know!