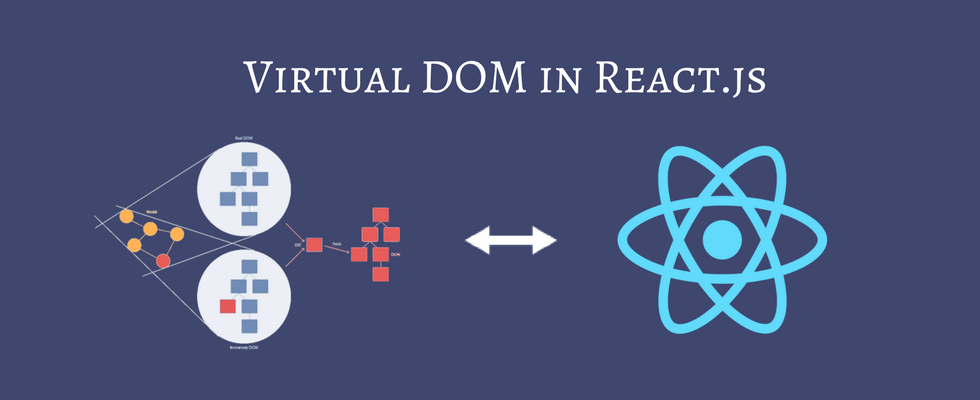
Do you know about DOM virtual in ReactJS?
The Virtual DOM in React.js is a concept that optimizes rendering performance by making updates more efficient. Here’s how it works and why it’s important:
1. What is the DOM?
- DOM (Document Object Model) represents the structure of a web page. It’s an interface that allows programs to interact with and modify the content, structure, and style of a website.
- Manipulating the real DOM (for example, when adding, removing, or updating elements) is slow because it requires recalculating styles, layout, and re-rendering the entire UI in the browser.
2. What is the Virtual DOM?
- The Virtual DOM is a lightweight in-memory representation of the actual DOM. It is a JavaScript object that React uses to keep track of UI changes in an efficient way.
- Instead of manipulating the real DOM directly, React updates the virtual DOM first.
3. How does it work?
- Initial Render: When a component is rendered for the first time, React builds a virtual DOM tree that mirrors the structure of the real DOM.
- Updates: When the state or props of a component change, React re-renders the virtual DOM and creates a new virtual DOM tree representing the updated state.
- Diffing: React compares the new virtual DOM with the previous version to identify which parts of the real DOM need to be changed. This process is called reconciliation or diffing.
- Batch Updates: After identifying the minimal set of changes, React updates only the necessary parts of the real DOM in a batch, which is much faster than updating the entire real DOM.
4. Why is the Virtual DOM fast?
- Efficient Reconciliation: By comparing the old and new virtual DOM trees, React can update only the parts of the UI that have changed, reducing unnecessary re-renders.
- Batching Updates: React can batch multiple updates and apply them all at once to minimize interactions with the real DOM.
- Minimized Layout Calculations: Since the real DOM is not manipulated directly until necessary, expensive layout recalculations are avoided.
5. Key Benefits
- Performance: Reduces the overhead of directly updating the real DOM, which can be slow, especially in complex applications.
- Declarative UI: Allows developers to describe how the UI should look based on the application’s state without worrying about manually managing updates to the DOM.
Example of Virtual DOM in Action
When you call setState
in React, the virtual DOM re-renders the updated component tree:
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
Here, when you click the button, only the specific part of the DOM (the text showing the count) will be updated, rather than the entire page being re-rendered, thanks to the virtual DOM diffing process.
Summary
- The Virtual DOM allows React to efficiently update the UI by comparing old and new virtual DOM trees and making only the necessary updates to the real DOM.
- This approach makes UI rendering faster, especially in large applications, by reducing the expensive operations needed to manipulate the real DOM.